Concepts that Every JavaScript Programmer Should Know
Javascript is a scripting programming language that allows the programmer to implement complex features on web pages. JS helps in displaying timely content updates, interactive maps, animated graphics, scrolling videos, etc. In short, the internet would be nothing with Javascript. In this post, I have covered some of the basic concepts in JS that every programmer should know.
1.Hoisting
It is a mechanism where functions and variables declarations are moved to the top of their scope before execution of the code. Hoisting allows the functions to be used in code before they have been declared.
For example,


Javascript only hoists declaration, it does not initialize. That means it doesn’t matter where the functions and variables have been declared, they are always moved to the top of their scope regardless of whether their scope is local or global.
2.Currying
Currying is a method to transform the functions with multiple arguments into multiple functions of a single argument in a sequence.
In simple words, a function takes the first argument and returns a new function simultaneously it takes the second function and returns a new function and then takes the third one and the process keeps on going until it fulfills all the arguments.
Example:
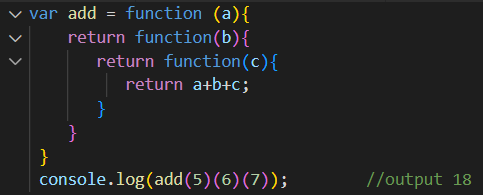
Currying helps to create high-order functions in event handling.
3. Polymorphism
Polymorphism is one of the key concepts for any object-oriented programming. Its use of it is to display a message in more than one form. It is the practice of designing the objects to share behaviors and override shared behaviors with specific ones and for it, polymorphism takes advantage of inheritance to make it happen.
Example:
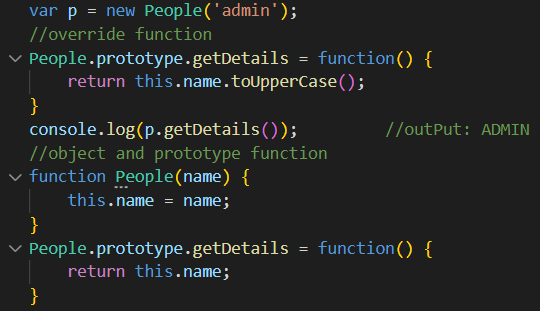
In the above program prototype-based method for people, the constructor function has to override by another prototype function to return the Name as uppercase.
We can override functions in different scopes, and it is also possible for method overloading. Even though Javascript doesn’t have a method of overloading but we can achieve it with the help of object-oriented programming.
4. Promises
A promise is an asynchronous function. The promise object represents the eventual completion of a synchronous operation of an asynchronous operation and its value. It can be used to avoid chaining callbacks.
A promise can be one of the below states:
pending: initial state, neither rejected nor fulfilled.
fulfilled: the operation was completed successfully.
rejected: the operation is failed.
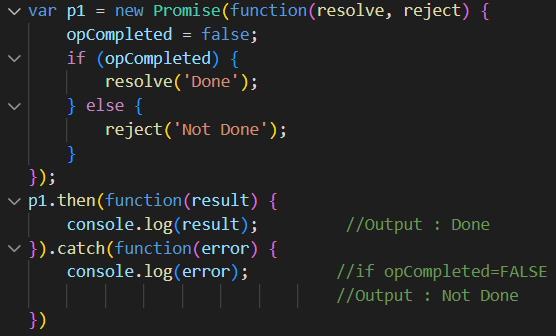
5. Async & Await
Async and Await make promises to write easily.
In JS asynchronous patterns can be handled in different versions like below:
in ES5, it is done by "Callback"
in ES6, it is done by "Promise"
in ES7, it is done by "async & await"
Many programmers have a misunderstanding about async & await, but they are promises only. Every async function returns a promise. The await can only be used inside an async function and everything that you will await will be a promise.
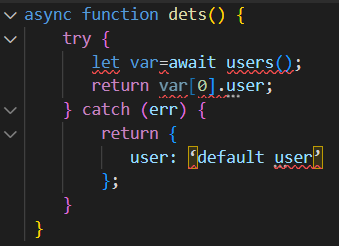
In order to know about async and await the programmer should have a proper understanding of the promises. If you can understand async & await, it will help you to implement functional programming easily and will increase the readability of code.
Please do let me know how you like this blog and also let me know if you’re interested in specific kind of blog, Thanks.